Programming errors are almost unavoidable, and debugging can take up a significant amount of time. As a programmer, you’ve probably used the print function countless times to debug your code.
While it’s a quick and easy way to see what’s going on in your program, it can become tedious and time-consuming as your programs get more complex.
Enter Icecream 🍦, a debugging tool that helps you debug your code more efficiently. In this blog post, we will discuss IceCream’s features, installation, and how to use it for debugging.
Table of Contents
What is Icecream 🍦?
Icecream is a Python library that makes print debugging more readable with minimal code.
Unlike print(), IceCream does not require the developer to write additional code or manually print out the variables. Instead, it automatically displays the information, making the debugging process much faster.
Icecream is a function that you can use to replace the print function in your code. It works by allowing you to specify the variables you want to debug and then print their values in a more readable and organized way.
In addition to its simplicity and readability, Icecream has some other benefits over using the print
function for debugging. For example, it’s faster and uses less memory, which can be especially important if you’re working with large datasets.
How Does IceCream Work?
IceCream works by analyzing the code and then displaying the results in a “breadcrumb” format. It will show the exact line of code that is causing the error and provide additional information such as the type of error, the variable’s value, and the object’s properties. This makes it easier to identify the root of the issue and allows developers to quickly fix the problem.
In addition, IceCream can also be used to debug multiple lines of code at once. This makes it easier for developers to find issues in more complex code and reduce the amount of time spent debugging.
Installing IceCream
To use Icecream, you’ll need to install it first using,
pip install icecream
Getting Started With IceCream – Inspect Variables
To inspect variables using Icecream, you’ll need to import it into your code and use it as a decorator on the function you want to debug.
For example, let’s say you have the following function:
def add(x, y):
return x + y
result = add(2, 3)
To use Icecream to inspect the variables in this function, you can do the following:
from icecream import ic
def add(x, y):
return x + y
ic(add(2, 3))
ic(add(4, 5))
In this example, the ic
decorator is applied to the add
function, which means that the variables x
and y
will be printed out when the function is called.
When you run this code, the output will look something like this:
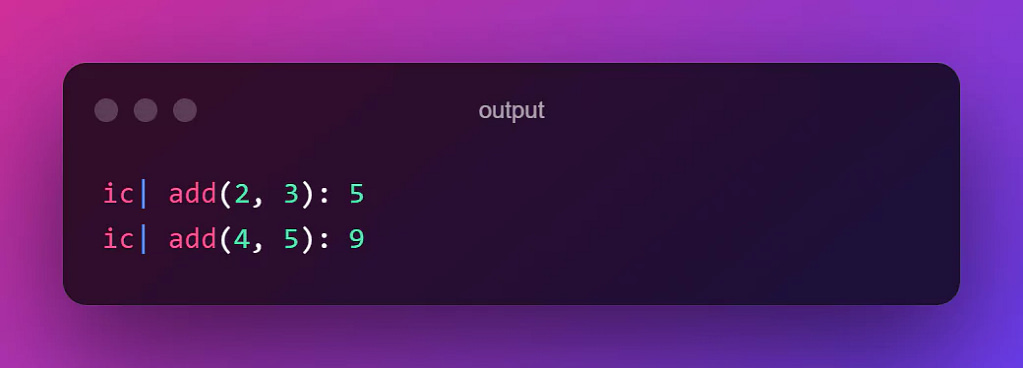
This output shows the values of the variables x
and y
as they are passed into the add
function, and the resulting value of the function call.
As you can see, the output is much more organized and readable than using the print
function.
You can also use Icecream to print out multiple variables at once, like this:
from icecream import ic
def multiply(x, y, z):
return x * y * z
ic(multiply(x=2, y=3, z=4))
ic(multiply(x=3, y=4, z=2))
The output will look like this:
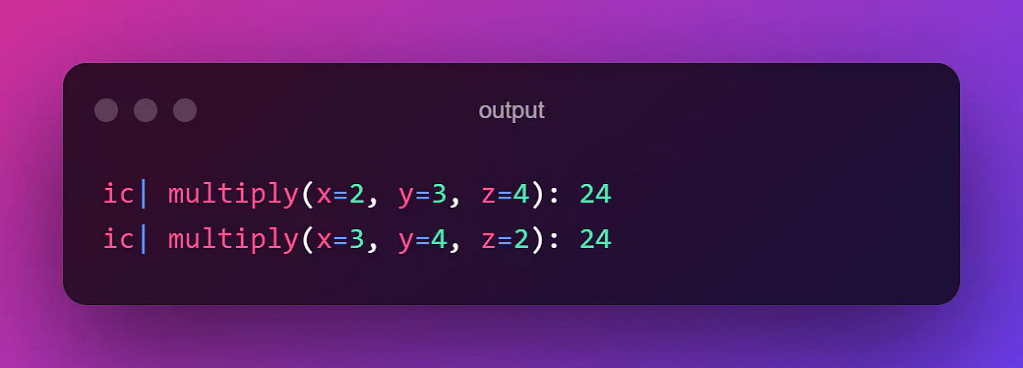
Overall, using Icecream to inspect variables can be a quick and effective way to understand what’s happening in your code. Give it a try and see how it can improve your debugging process.
Inspect a Dictionary using IceCream
To access a dictionary using Icecream, you can simply use the ic
function and pass the function which contains the dictionary and then access the dictionary in the usual way.
For example, consider the following code:
from icecream import ic
my_dict = {'a': 1, 'b': 2, 'c': 3}
ic(my_dict['a'])
ic(my_dict['b'])
In this example, the ic
function, output the key
and the value pair will be printed out when the function is called.
The output will look something like this:
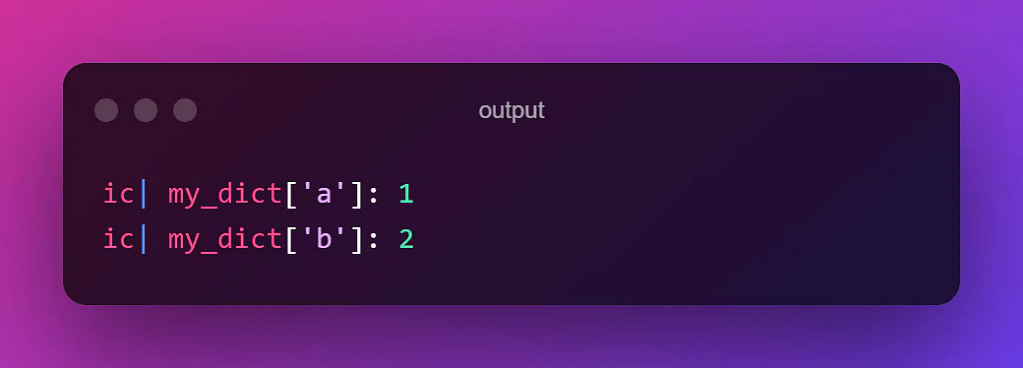
Inspect Attributes of an Object using IceCream
To access the attributes of an object using Icecream, you can use the ic
a decorator on the function that contains the object and then accesses the object’s attributes in the usual way.
For example, consider the following code:
from icecream import ic
class MyClass:
def __init__(self, x, y):
self.x = x
self.y = y
obj = MyClass(1, 2)
In this example, the ic decorator is applied to the get_attributes function, which means that the value of the variable obj
will be printed out when the function is called.
ic(obj.x, obj.y)
The output will look something like this:
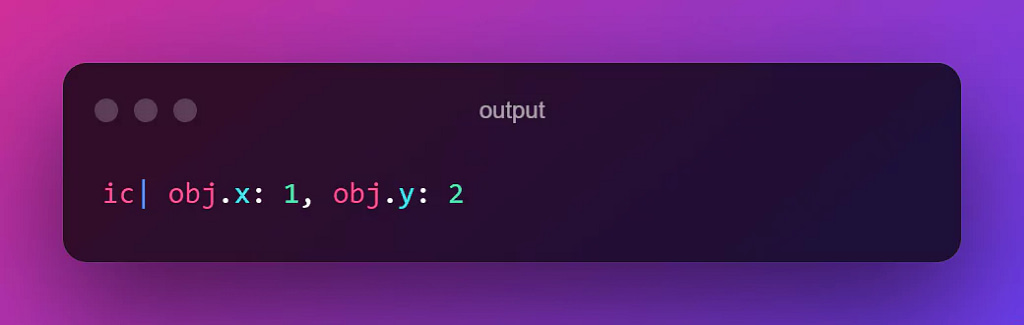
Debug in If-Condition using IceCream
To debug an if-condition using Icecream, you’ll need to use the ic
a function containing the if-condition. It will allow you to print out the values of variables at each step of the function’s execution.
Here’s an example of how you might use Icecream to debug an if-condition:
from icecream import ic
def check_even(x):
if x % 2 == 0:
ic()
else:
ic()
check_even(4)
In this example, the ic
the function is applied to the check_even
function, which means that the value of the variable x
will be printed out when the function is called.
The output will look something like this:
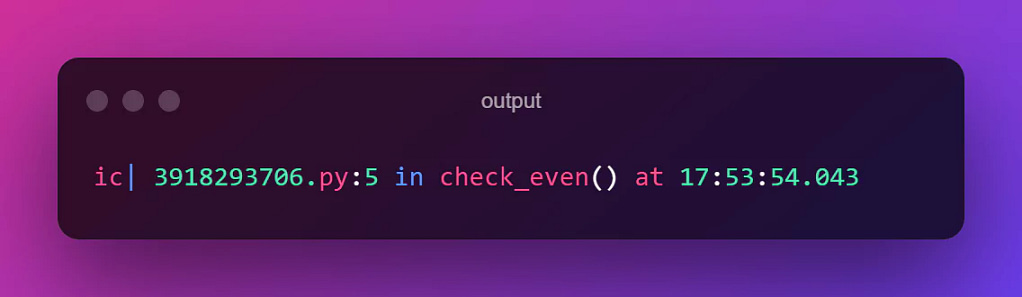
Overall, using Icecream to debug if-conditions can be a quick and effective way to understand what’s happening in your code. Give it a try and see how it can improve your debugging process.
Customizing IceCream Output
If you want to add a custom prefix, such as the time the code was executed, to your print statements using Icecream, you can do so by specifying the configureOutput
function as passing time_format as an argument.
from datetime import datetime
from icecream import ic
import time
def time_format():
return f'{datetime.now()}| debug |> '
ic.configureOutput(prefix=time_format)
for _ in range(3):
time.sleep(1)
ic('Hello World')
If you execute this code it will be going to show the execution time in the output.
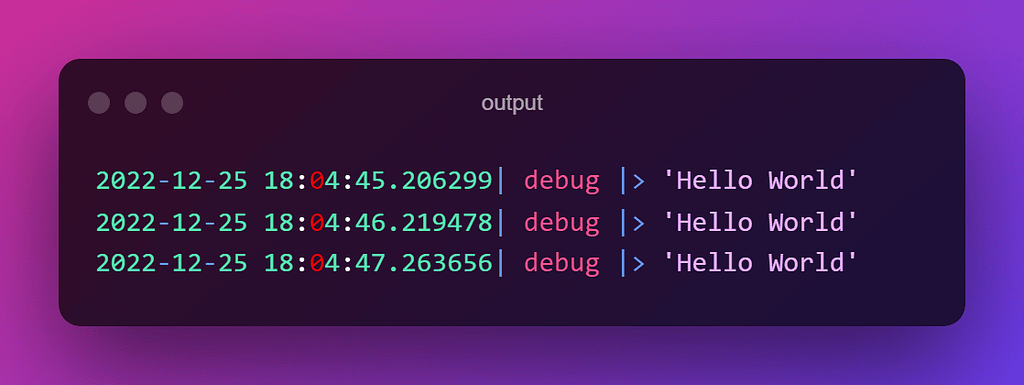
Now if you want more context for the output. You might also want to know the line number and file name from where the code was executed from. We can easily get the context of the code by adding includeContext=True as an argument in ic.configureOutput().
from icecream import ic
ic.configureOutput(includeContext=True)
def check_even(x):
if x % 2 == 0:
ic()
else:
ic()
ic(check_even(4))
You can see the output below,
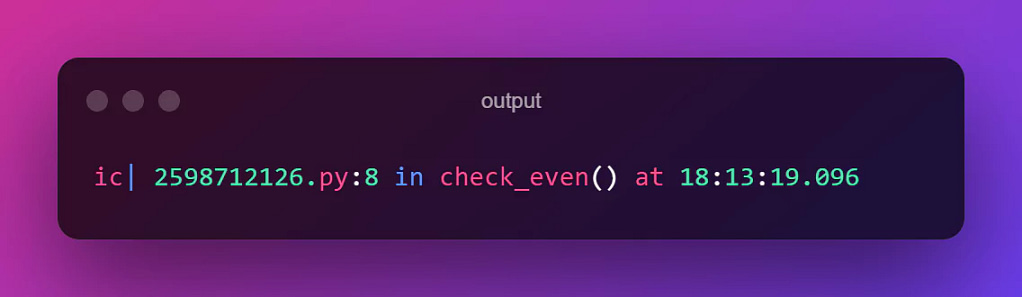
Use IceCream Project Wide
If you are working on a project where you have hundreds of files then importing the ice cream library in every file will be a nightmare. There is an easy way around this problem.
You can make this method available project-wide by importing the install() module from ice cream in the root file, as shown below:
## main_file.py
from icecream import install
install()
from help_file import func
func(2)
## help_file.py
def func(num):
ic(num)
return 2*num
With this approach install() and ic() will be available in all the project files.
Deleting IceCream after debugging
Once you completed debugging your code. You can remove all the unnecessary debugging statements. You can simply remove the ic function calls from your code. This will prevent Icecream from printing out the values of variables as your code runs.
For example, consider the following code:
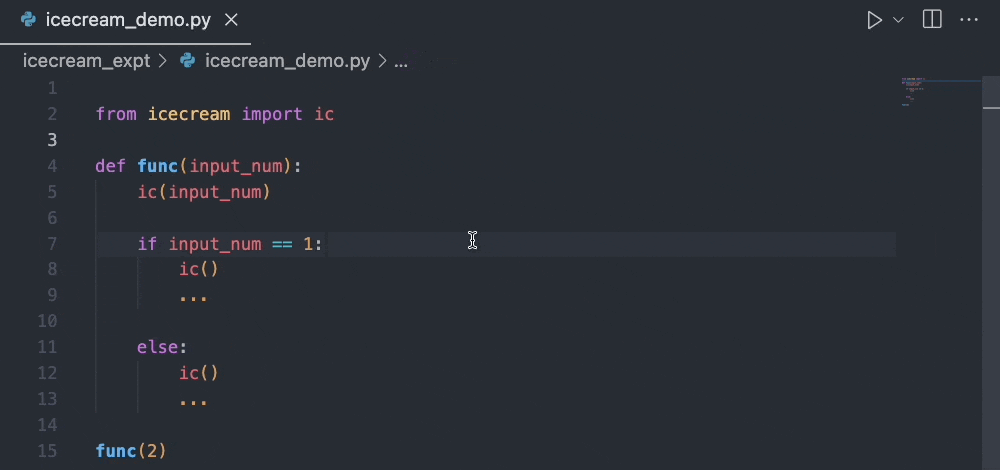
You can just search for pattern “ic(” in your IDE and remove all the statements.
Note: If you want to disable IceCream for short period and might need it later on then you can use ic.disable(). This prevents ic() from printing any output. When you want to use it again you can enable it by ic.enable().
Why Is IceCream So Valuable?
IceCream is incredibly valuable for Python developers because it helps speed up the debugging process. By providing detailed information about the code and eliminating the need to manually print out variables, it can save developers hours of time and make debugging much easier.
In addition, IceCream is also very easy to use and understand. Even developers who are new to Python can quickly learn how to use it and start debugging their code right away.
Conclusion
Using Icecream to inspect the execution of your code can be more efficient and effective than using the print
function for several reasons.
First, Icecream allows you to specify the variables you want to debug, rather than having to manually print them out with the print
function. This can save you time and make it easier to focus on the specific variables you’re interested in.
Second, Icecream prints out the values of variables in a more organized and readable way. Instead of having to scroll through a long list of print
statements, you can see all the relevant variables and their values at a glance.
Third, Icecream allows you to specify the names of the variables you want to print, which can be especially helpful if you’re working with large and complex programs. This can make it easier to understand what’s happening in your code, especially if you’re working with multiple developers or on a team.
Overall, using Icecream can make debugging your code faster and more efficient, which can save you time and frustration. If you’re tired of using the print
function to debug your code, give Icecream a try, and see how it can improve your workflow.
So next time you’re debugging your code, consider using Icecream instead of the print
function. You’ll save time and frustration, and your code will be easier to read and understand.
Thanks for reading! I hope you this debugging tool will save a lot of your time while finding bugs.
If you like this article and wish to connect with me follow me on Linkedin.
Be curious, keep learning and stay willing to learn new things until we meet next time!